OrderCloud Fundamentals - Part 1
The OrderCloud SDK
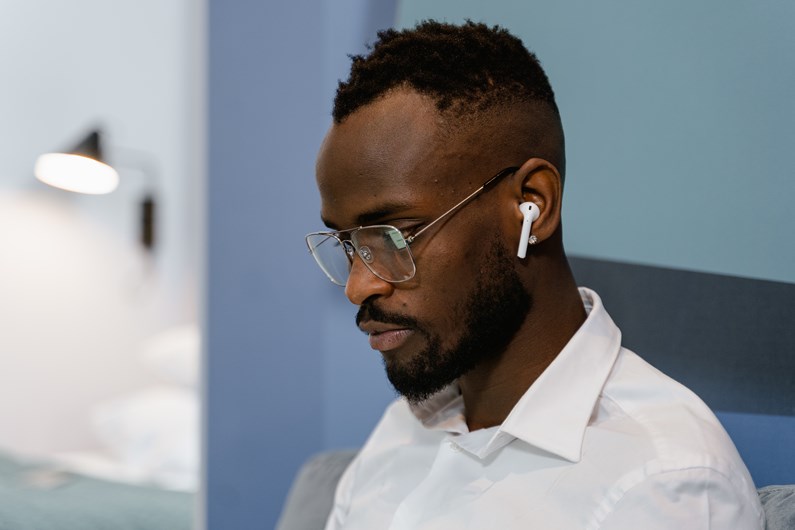
februari 28, 2022
A while ago Sitecore acquired Four51 and their product OrderCloud. OrderCloud is a SaaS eCommerce platform, capable of handling complex B2B, B2C and even B2X commerce scenarios. Let's discover some of the development fundamentals of OrderCloud.
OrderCloud is an API-first and headless platform, so developing against it is very different compared to traditional Sitecore development. You can no longer alter the application’s behavior by adding in your own code and/or overwriting functionalities of the base application. Instead you need to build your own application while using their APIs to enrich the functionalities you offer in your webshop. But how do you do that and where do you start?
In this series of posts I will be going over some of the fundamentals of working with Sitecore OrderCloud.
We will be looking at the different tools and libraries OrderCloud provides us with in order to build our applications. The series is split into 3 different parts. In parts 1 and 2 we will be looking at different libraries we can use in our application and in the final part we will put it all together to define what the architecture of an OrderCloud based application might look like.
- OrderCloud SDK
- OrderCloud Catalyst
- Defining an architecture
So, in this first part we will be taking a look at the OrderCloud SDK. The SDK comes in different languages, as part of this series we will be working with the .NET stack, so we will also be looking at the .NET SDK.
The .NET SDK is available as a NuGet package by the name "OrderCloud.SDK" and works with all the latest .NET versions (I used .NET 6.0).
This SDK is a library you will probably always need in order to build any OrderCloud based application.
As we know, OrderCloud is a headless commerce platform, meaning that the only way of communicating with it is through APIs. The SDK makes it easier for you to perform API requests to OrderCloud by giving you a structured way of managing connections to OrderCloud and providing an typed approach for performing those requests.
The OrderCloudClient
The .NET version of the SDK uses 'Clients' to perform API requests and store access tokens.
The first thing you want to do is create one of those clients:
using OrderCloud.SDK; var client = new OrderCloudClient(new OrderCloudClientConfig { ClientId = "my-client-id", // client credentials grant flow: ClientSecret = "my-client-secret" // OR password grant flow: Username = u, Password = p, Roles = new[] { ApiRole.OrderAdmin } });
Each instance of a client automatically requests an access token on creation and stores it in it's context. As you can see in above code snippet there are 2 ways of authenticating using these clients, using a username-password combination or using a secret.
This can be used to create a client for each user which authenticates with your application, but if you don't want to do that you can also use the impersonation approach, which is the approach I would choose when building browser based applications.
With the impersonating approach you can create a single client using a ClientSecret for initial authentication. After that you can specify an access token which should be used for the API requests to OrderCloud. This access token you could get from your client-side application, so you would still need the JavaScript SDK or a direct API integration in your frontend application.
Once you have a client, you can use it to perform API requests.
var orders = await client.Orders.ListAsync(OrderDirection.Incoming, filters: new { Status = OrderStatus.Open });
As you can see in above example, the client provides a structure of methods and objects to perform API requests. This structure is based on the structure OrderCloud has put in their APIs, so for each category of APIs there is an instance of an interface in the OrderCloudClient object.
Each of these interfaces has an implementation for each API request part of that category. For structured objects like Products, Categories and Orders that includes CRUD like implementations.
So, instead of having to build your own client and maintain HttpClients, you can use these OrderCloudClients to connect to the OrderCloud APIs in a much easier way.
Extended Properties
Another big advantage of using this SDK is that you can use Strongly Typed Extended Properties.
Extended Properties, or in short 'xp' (yes, this might be confusing when you come from a Sitecore background), is a feature in OrderCloud which you can use to extend their data models. On many different models in OrderCloud you will see a property 'xp', for which you can specify any value to. This can be a simple flat object structure as well as multiple levels of objects.
These extended properties can be used on models like the Product and Category models.
There are two options in the .NET SDK on how you can specify this xp property. The first option is to specify the dynamic object directly on the instance of the model. For example, on the OrderCloud.SDK.Product model we can specify the xp property as a dynamic object:
var product = new OrderCloud.SDK.Product(); product.xp = new { Brand = "Microsoft" };
The second option is to set it using a strongly typed object. When you use the OrderCloud client to perform API requests to OrderCloud, you can in some cases also specify the Type of object the API request should send/return.
If you look at the interface for the Product APIs, then it has this Get method:
Task GetAsync(string productID, string accessToken = null) where TProduct : Product;
As you can see, you can specify a TProduct when calling this method. TProduct needs to be based on OrderCloud.SDK.Product. We can use OrderCloud.SDK.Product as Type for these requests. Txp in this case can be any type of object and is assigned to the 'xp' property of the Product:
// Type parameters: // Txp: // Specific type of the xp property. If not using a custom type, use the non-generic // Product class instead. public class Product : Product { // Summary: // Container for extended (custom) properties of the product. public new Txp xp { get { return GetProp("xp"); } set { SetProp("xp", value); } } }
So, for Txp we can create a new model which specifies the properties our extended properties object should have, for example:
public class ProductXp { public string Brand { get; set; } }
Now we can use these types to make the Get request to the OrderCloud APIs. The SDK will then deserialize the JSON response from OrderCloud and convert it to the type we specify.
var result = await client.Products.GetAsync<Product>(productId);
This same principle is applied to the PartialProduct model:
public class PartialProduct : PartialProduct
Alternatives
In this blogpost we looked at the .NET version of the OrderCloud SDK, but there are alternatives available if you don't want to build the integration in .NET.
One of the alternatives is to use the JavaScript SDK, which is very similar to the .NET version. This JavaScript version offers the same functionalities and typed approach on performing API requests. It does not contain the same setup with an OrderCloudClient, but it looks a lot alike. The documentation of that SDK shows how easy it is to use it.
The other alternative is to use the OrderCloud APIs directly. The APIs are built based on the Open API standards, meaning that you can easily import the API specifications in a tool like Swagger or Postman in order to play around with the APIs directly.
So, now you know how you can use the OrderCloud SDKs to build an application for yourself. But OrderCloud offers more than just SDKs for their APIs.
In the next post we will be looking at OrderCloud Catalyst, a library of helper functionalities which will help you build your own Middleware application on .NET.